원본: JavaScript Substring Method
이번 포스트에서는 자바스크립트의 substring() 메소드를 효과적으로 사용하는 방법에 대해 알아보겠습니다. 여기서는 메소드의 작동 방식을 알아보기위해 예제와 패턴, 특이점 등을 확인해 보겠습니다.
일반적으로 substring() 메소드는 문자열의 일부를 추출하고 저장할 때 사용하는 String 객체의 메소드입니다. 추출하는 동안 원본 문자열은 그대로 유지되며 대상 부분만 새 문자열로 리턴됩니다.
문자열의 특정 부분을 가져올 때 사용하기 좋습니다. 이 특정 문자열은 문자열의 시작, 중간, 끝 부분이 될 수 있습니다.
Table of Contents
자바스크립트 substring 메소드
이 메소드는 startIndex, endIndex 두 개의 매개변수를 받습니다. startIndex는 필수 매개변수이고, endIndex는 추출할 문자열의 끝을 지정하기 위한 옵셔널 값 입니다.
substring 메소드 문법 구문
다음의 문법 구문을 사용할 수 있습니다:
substring(startIndex);
substring(startIndex, endIndex);
startIndex는 하위 문자열 추출이 시작되는 지점을 나타냅니다. 이는 결과 값에 포함되는 인덱스 입니다.
endIndex는 추출 종료 지점을 나타냅니다. 단, endIndex의 값은 결과 값에서 제외됩니다. 이는 endIndex 앞의 문자에서 추출을 종료한다는 의미입니다.
원본 문자열 끝의 하위 문자열 가져오기
startIndex만 메소드에 전달하면 startIndex 부터 원본 문자열의 끝까지를 리턴합니다.
const mnemonic = "Please, send, cats, monkeys, and, zebras, in, large, cages, make, sure, padlocked.";
console.log(mnemonic.substring(14));
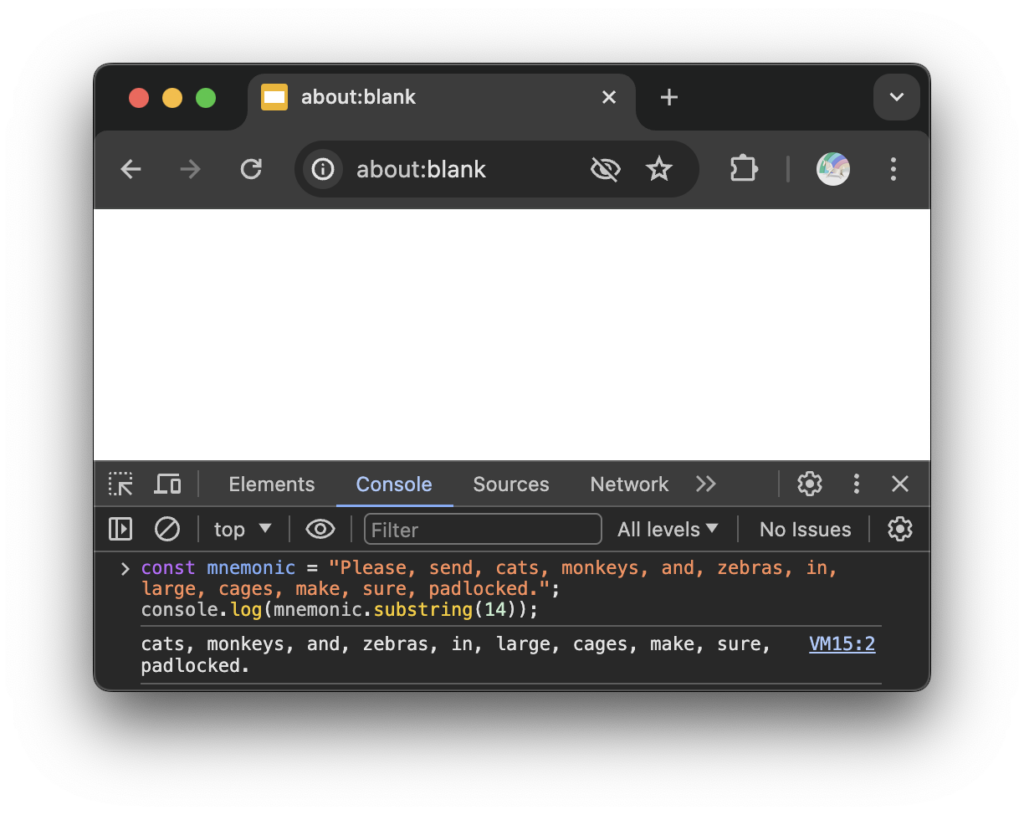
개발자 도구에서 console.log() 메소드를 사용하여 예제를 테스트해보면 위의 화면과 같은 결과를 얻을 수 있습니다.
원본 문자열 중간의 하위 문자열 가져오기
startIndex와 endIndex를 모두 매개변수로 전달하면 startIndex <= str < endIndex
범위의 하위 문자열을 얻습니다.
const mnemonic = "Please, send, cats, monkeys, and, zebras, in, large, cages, make, sure, padlocked.";
console.log(mnemonic.substring(14, 27));
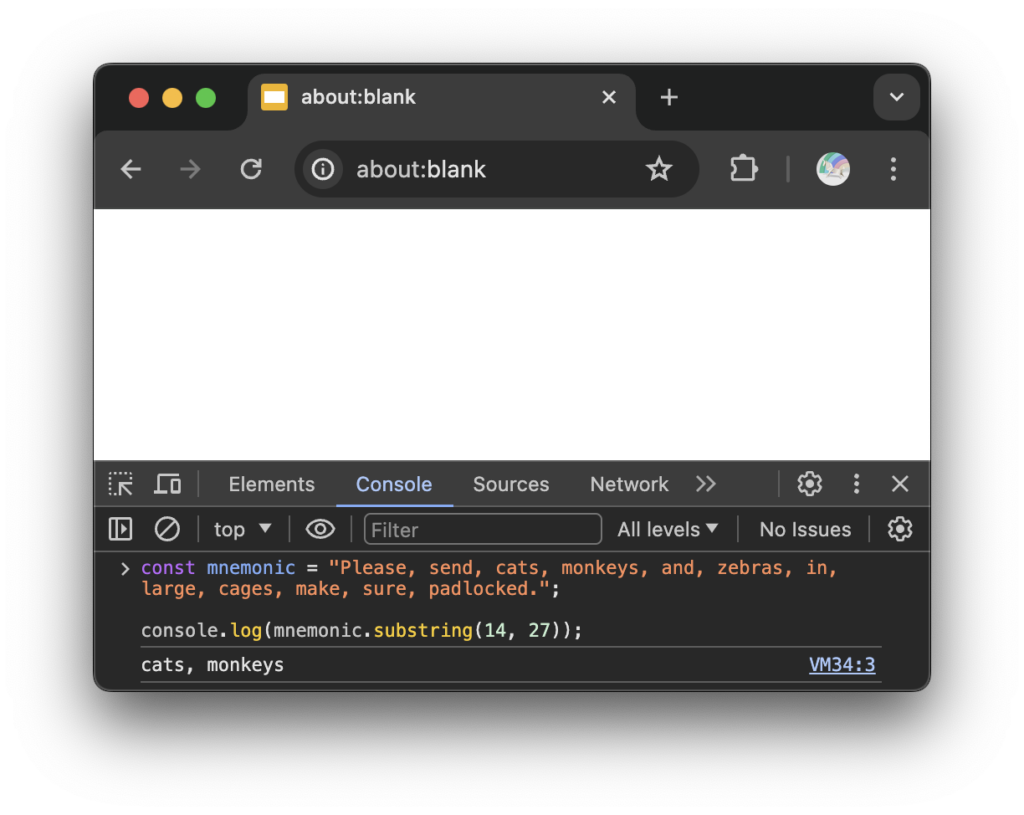
이는 endIndex 앞에서 끝나는 하위 문자열을 가져옵니다.
원본 문자열의 앞쪽 하위 문자열 가져오기
원본 문자열의 시작 부분 부터 가져오고 싶은 경우 startIndex 값은 0 이어야 합니다.
const mnemonic = "Please, send, cats, monkeys, and, zebras, in, large, cages, make, sure, padlocked.";
console.log(mnemonic.substring(0, 27));
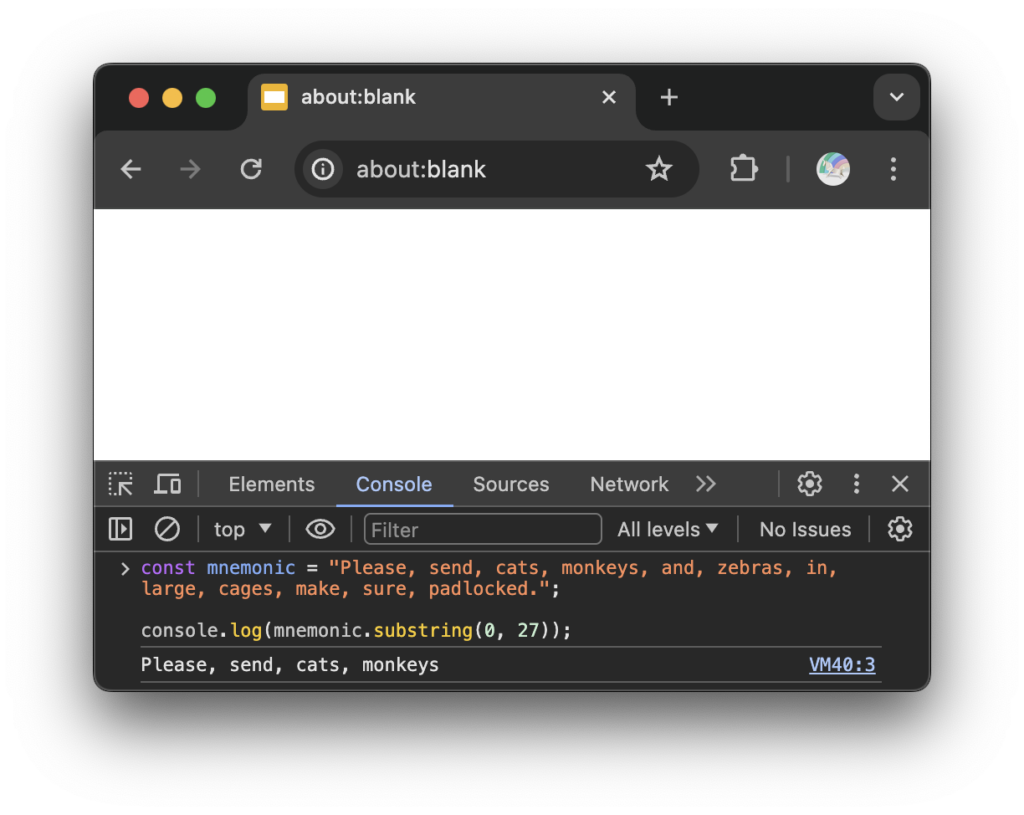
startIndex를 0으로 하고 endIndex를 n으로 생각하면 원본 문자열의 첫 n 개의 문자를 가져오는 것과 마찬가지 입니다.
const mnemonic = "Please, send, cats, monkeys, and, zebras, in, large, cages, make, sure, padlocked.";
// First 12 characters
console.log(mnemonic.substring(0, 12)); // "Please, send"
// First 18 characters
console.log(mnemonic.substring(0, 18)); // "Please, send, cats"
// First 27 characters
console.log(mnemonic.substring(0, 27)); // "Please, send, cats, monkeys"
// First 70 characters
console.log(mnemonic.substring(0, 70)); // "Please, send, cats, monkeys, and, zebras, in, large, cages, make, sure"
마지막 n 개의 문자 가져오기
length 속성을 startIndex에 사용하여 마지막 n 개의 문자를 얻을 수 있습니다.
const mnemonic =
"Please, send, cats, monkeys, and, zebras, in, large, cages, make, sure, padlocked.";
// Last 9 characters
console.log(mnemonic.substring(mnemonic.length - 9)); // "padlocked."
// Last 15 characters
console.log(mnemonic.substring(mnemonic.length - 15)); // "sure, padlocked."
substring() 메소드의 특이한 매개변수
startIndex가 endIndex 보다 큰 경우와 음수 인덱스가 전달된 경우 어떻게 동작하는지 확인해 보겠습니다.
startIndex > endIndex
startIndex 값이 더 큰 경우 substring() 메소드는 두 값을 서로 바꿔서 결과 값을 리턴합니다.
const mnemonic = "Please, send, cats, monkeys, and, zebras, in, large, cages, make, sure, padlocked.";
console.log(mnemonic.substring(6, 0));
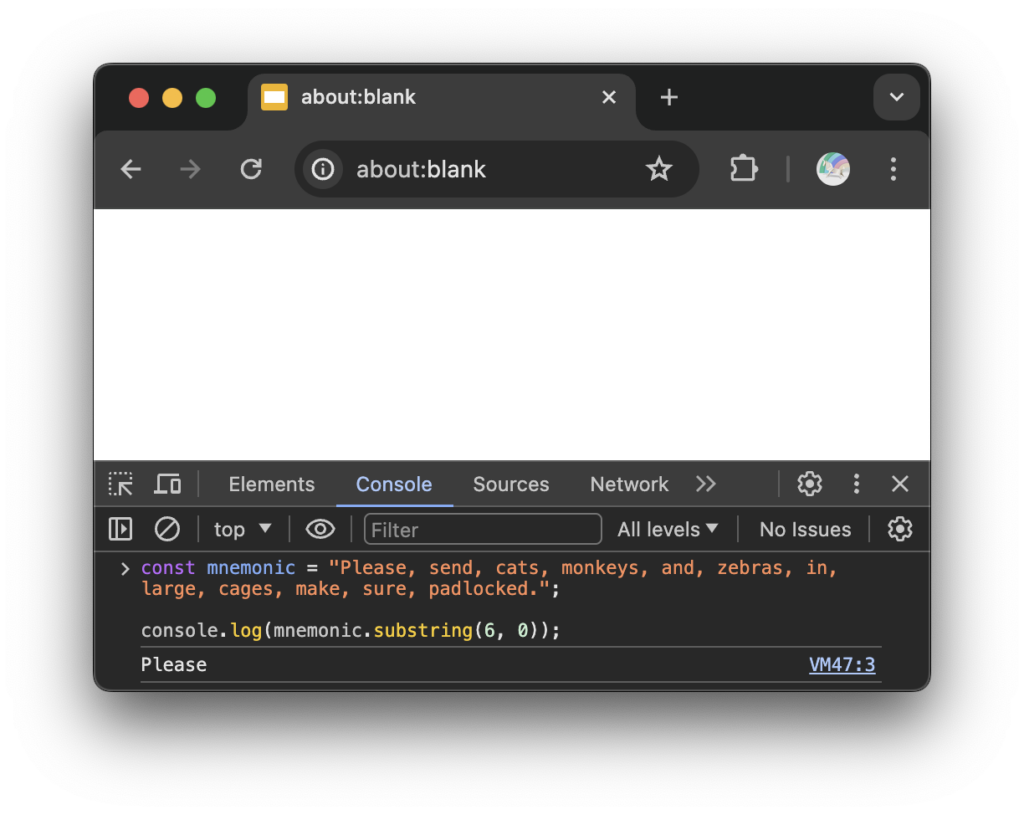
음수 매개변수
매개변수로 전달된 값이 음수인 경우 해당 값을 0으로 변경합니다.
const mnemonic = "Please, send, cats, monkeys, and, zebras, in, large, cages, make, sure, padlocked.";
console.log(mnemonic.substring(-1, 6)); // "Please"
console.log(mnemonic.substring(-1, -6)); // ""
console.log(mnemonic.substring(12, -6)); // "Please, send"
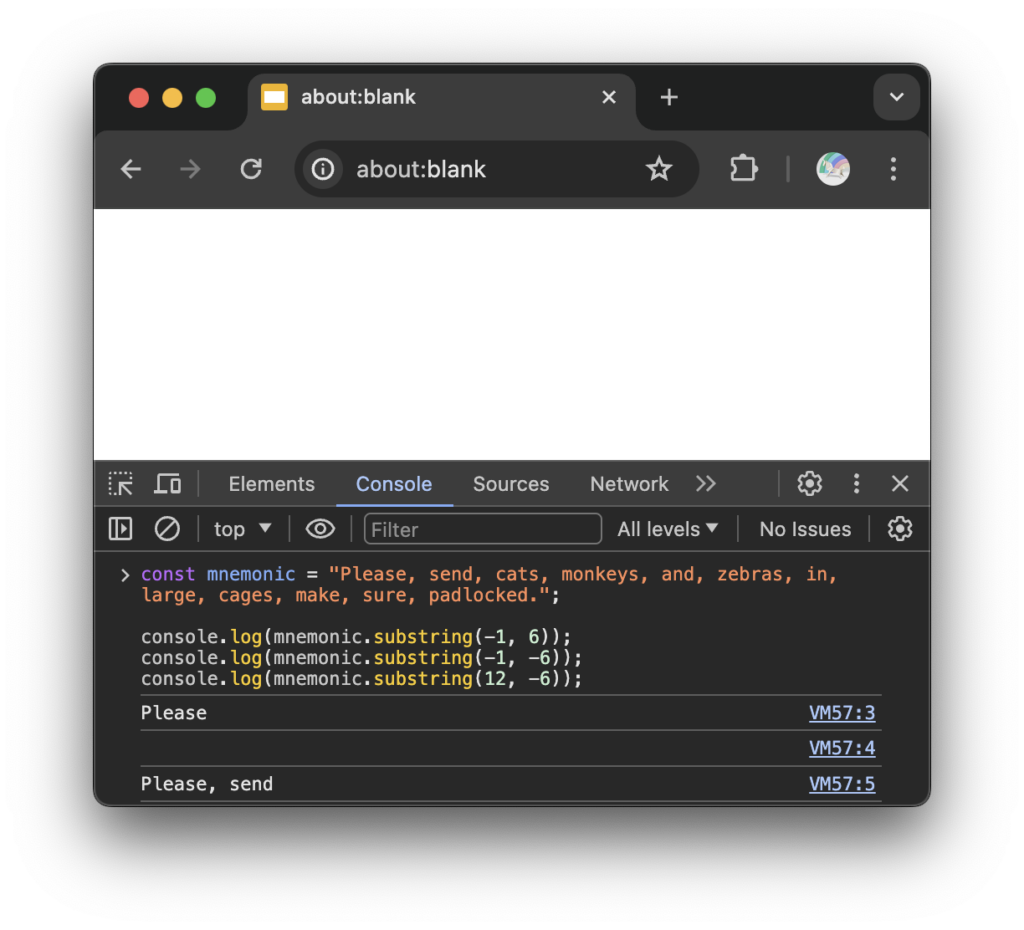
마지막 호출에서는 startIndex가 더 크므로 두 매개변수의 교환도 진행됩니다.
substring() 메소드와 slice() 메소드 비교
substring() 메소드와 slice() 메소드는 모두 하위 문자열을 추출하는 메소드지만 구현에는 미묘한 차이가 있습니다.
예를 들어 slice() 메소드에서는 startIndex 값이 endIndex 보다 더 큰 경우 매개변수 교환을 하지 않습니다.
const mnemonic = "Please, send, cats, monkeys, and, zebras, in, large, cages, make, sure, padlocked.";
console.log(mnemonic.substring(6, 0)); // "Please"
console.log(mnemonic.slice(6, 0)); // ""
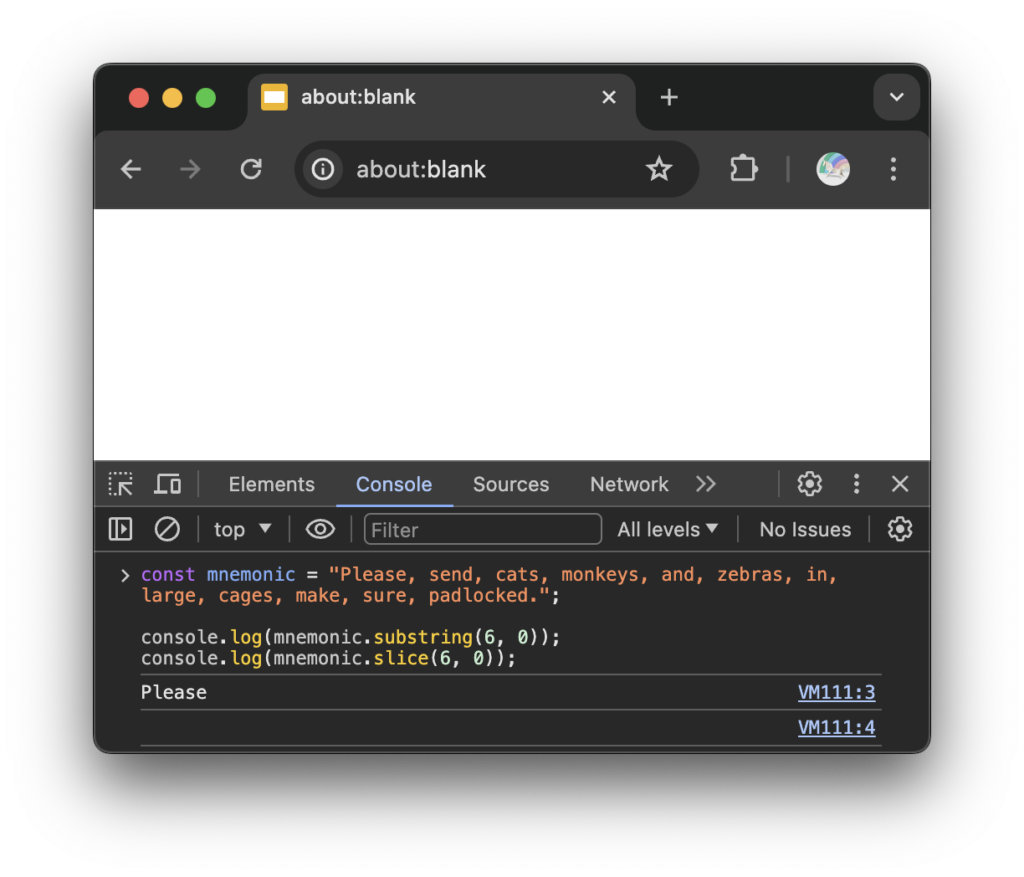
slice() 메소드는 빈 문자열을 리턴했습니다.
또 다른 차이점은 매개변수 값이 음수일 때 입니다.
const mnemonic = "Please, send, cats, monkeys, and, zebras, in, large, cages, make, sure, padlocked.";
console.log(mnemonic.substring(18, -12)); // "Please, send, cats"
console.log(mnemonic.slice(20, -12)); // "monkeys, and, zebras, in, large, cages, make, sure"
console.log(mnemonic.substring(-12, 18)); // "Please, send, cats"
console.log(mnemonic.slice(-12, 18)); // ""
console.log(mnemonic.substring(-16, -12)); // ""
console.log(mnemonic.slice(-16, -12)); // "sure"
매개변수가 음수인 경우 문자열의 끝에서 부터 인덱스를 찾게 됩니다.
정리
이 포스트에서는 자바스크립트의 substring() 메소드에 대해 알아봤습니다.
메소드에 startIndex와 endIndex를 전달함으로 하위 문자열을 가져올 수 있고 특정 패턴을 통해 원본 문자열의 시작, 중간, 끝 부분을 지정할 수 있습니다.